Bài giảng Phát triển phần mềm nguồn mở - Bài 7: Route, views, blade templates - Nguyễn Hữu Thể
Routing
− Basic Routing
− Route Parameters
• Required Parameters
• Optional Parameters
• Regular Expression Constraints
− Named Routes
− Route Groups
• Middleware
• Namespaces
• Sub-Domain Routing
• Route Prefixes
− Route Model Binding
• Implicit Binding
• Explicit Binding
− Form Method Spoofing
− Accessing The Current Route
Bạn đang xem 20 trang mẫu của tài liệu "Bài giảng Phát triển phần mềm nguồn mở - Bài 7: Route, views, blade templates - Nguyễn Hữu Thể", để tải tài liệu gốc về máy hãy click vào nút Download ở trên
Tóm tắt nội dung tài liệu: Bài giảng Phát triển phần mềm nguồn mở - Bài 7: Route, views, blade templates - Nguyễn Hữu Thể
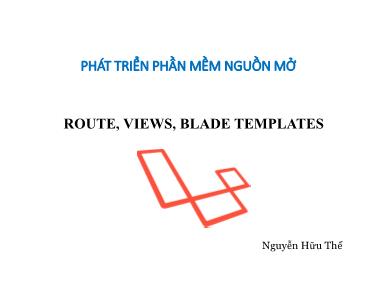
PHÁT TRIỂN PHẦN MỀM NGUỒN MỞ ROUTE, VIEWS, BLADE TEMPLATES Nguyễn Hữu Thể Routing − Basic Routing − Route Parameters • Required Parameters • Optional Parameters • Regular Expression Constraints − Named Routes − Route Groups • Middleware • Namespaces • Sub-Domain Routing • Route Prefixes − Route Model Binding • Implicit Binding • Explicit Binding − Form Method Spoofing − Accessing The Current Route 2 Routing Image from: 3 Basic Routing − Laravel routes: providing a very simple and expressive method of defining routes: Route::get ('/', function () { return view('welcome'); } ); − For most applications, you will begin by defining routes in your routes/web.php file. − Test: 4 Basic Routing Route::get ( 'foo', function () { return 'Hello World'; } ); − Test: 5 Available Router Methods − The router allows you to register routes that respond to any HTTP verb: Route::get($uri, $callback); Route::post($uri, $callback); Route::put($uri, $callback); Route::patch($uri, $callback); Route::delete($uri, $callback); Route::options($uri, $callback); 6 Route Parameters Route::get ( 'foo', function () { return 'Hello World'; } ); Route::get ( '/', function () { return 'Hello World'; } ); Route::post ( 'foo/bar', function () { return 'Hello World'; } ); Route::put ( 'foo/bar', function () { // } ); Route::delete ( 'foo/bar', function () { // } ); 7 Responds to multiple HTTP − Using the match method. Route::match ( [ 'get','post' ], '/', function () { return 'Hello World'; } ); − Or, register a route that responds to all HTTP verbs using the any method. Route::any ( 'foo', function () { return 'Hello World'; } ); 8 Route Parameters − You may need to capture a user's ID from the URL. You may do so by defining route parameters: Route::get ( 'hello/{name}', function ($name) { return 'Hello ' . $name; } ); 9 Route Parameters − You may define as many route parameters as required by your route: Route::get ( 'posts/{post}/comments/{comment}', function ($postId, $commentId) { // } ); − Note: • Route parameters are always encased within {} braces and should consist of alphabetic characters. • Route parameters may not contain a - character. Use an underscore (_) instead. 10 Optional Parameters − Placing a ? mark after the parameter name. Make sure: a default value Route::get ( 'user/{name?}', function ($name = null) { if ($name == null) //Response to else //Response to } ); Route::get ( 'user/{name?}', function ($name = 'John') { return $name; } ); 11 Regular Expression Constraints − Constrain the format of your route parameters using the where method on a route instance. Route::get ( 'user/{name}', function ($name) { return $name; } )->where ( 'name', '[A-Za-z]+' ); Route::get ( 'user/{id}', function ($id) { return $id; } )->where ( 'id', '[0-9]+' ); Route::get ( 'user/{id}/{name}', function ($id, $name) { return $id . ' ' . $name; } )->where ( [ 'id' => '[0-9]+','name' => '[a-z]+' ] ); 12 Regular Expression Constraints 13 Global Constraints − A route parameter to always be constrained by a given regular expression, use the pattern method. − Define these patterns in the boot method of your RouteServiceProvider: app\Providers\RouteServiceProvider.php public function boot(){ Route::pattern('id', '[0-9]+'); parent::boot(); } − Once the pattern has been defined, it is automatically applied to all routes using that parameter name: Route::get('user/{id}', function ($id) { // Only executed if {id} is numeric... }); 14 Named Routes − The convenient generation of URLs or redirects for specific routes. − name method: Route::get ( 'user/profile', function () { // } )->name ( 'profile' ); − You may also specify route names for controller actions: Route::get('user/profile', 'UserController@showProfile')->name('profile'); 15 Generating URLs To Named Routes − Use the route's name when generating URLs or redirects via the global route function: // Generating URLs... $url = route('profile'); // Generating Redirects... return redirect()->route('profile'); − If the named route defines parameters, you may pass the parameters as the second argument to the route function. Route::get('user/{id}/profile', function ($id) { // })->name('profile'); $url = route('profile', ['id' => 1]); 16 Route Groups − Share route attributes: • middleware • Namespaces • Sub-Domain Routing • Route Prefixes − Shared attributes are specified in an array format as the first parameter to the Route::group() method. 17 Middleware − To assign middleware to all routes within a group, you may use the middleware key in the group attribute array. Route::group ( [ 'middleware' => 'auth' ], function () { Route::get ( '/', function () { // Uses Auth Middleware } ); Route::get ( 'user/profile', function () { // Uses Auth Middleware } ); } ); 18 Namespaces − Use-case for route groups is assigning the same PHP namespace to a group of controllers using the namespace parameter in the group array: Route::group ( [ 'namespace' => 'Admin' ], function () { // Controllers Within The //"App\Http\Controllers\Admin" Namespace } ); − Default: the RouteServiceProvider includes your route files within a namespace group, allowing you to register controller routes without specifying the full App\Http\Controllers namespace prefix. 19 Sub-Domain Routing − Route groups may also be used to handle sub-domain routing. − The sub-domain may be specified using the domain key on the group attribute array: Route::group(['domain' => '{account}.myapp.com'], function () { Route::get('user/{id}', function ($account, $id) { // }); }); 20 Route Prefixes − The prefix group attribute may be used to prefix each route in the group with a given URI. • For example, you may want to prefix all route URIs within the group with admin. Route::group ( [ 'prefix' => 'admin' ], function () { Route::get ( 'users', function () { // Matches The "/admin/users" URL } ); } ); 21 Implicit Binding − Laravel automatically resolves Eloquent models defined in routes or controller actions whose variable names match a route segment name. For example: Route::get('api/users/{user}', function (App\User $user) { return $user->email; }); − In this example: • Since the Eloquent $user variable defined on the route matches the {user} segment in the route's URI, • Laravel will automatically inject the model instance that has an ID matching the corresponding value from the request URI. 22 Customizing The Key Name − Model binding to use a database column other than id when retrieving a given model class: • Override the getRouteKeyName method on the Eloquent model: // Get the route key for the model. // @return string public function getRouteKeyName(){ return 'slug'; } 23 Explicit Binding − Use the router's model method. • In the boot method of the RouteServiceProvider class: public function boot(){ parent::boot(); Route::model('user', App\User::class); } − Next, define a route that contains a {user} parameter: Route::get ( 'profile/{user}', function (App\User $user) { // } ); − Since we have bound all {user} parameters to the App\User model, • A User instance will be injected into the route. • For example, a request to profile/1 will inject the User instance from the database which has an ID of 1. 24 Customizing The Resolution Logic − Use your own resolution logic • Use the Route::bind method. public function boot(){ parent::boot(); Route::bind('user', function ($value) { return App\User::where('name', $value)->first(); }); } 25 Form Method Spoofing − HTML forms do not support PUT, PATCH or DELETE actions. • So, when defining PUT, PATCH or DELETE routes that are called from an HTML form, you will need to add a hidden _method field to the form. • The value sent with the _method field will be used as the HTTP request method. − Use the method_field helper to generate the _method input: {{ method_field('PUT') }} 26 Accessing The Current Route − To access information about the route handling the incoming request. $route = Route::current (); $name = Route::currentRouteName (); $action = Route::currentRouteAction (); 27 Views 1. Creating Views 2. Passing Data To Views 3. Sharing Data With All Views 4. View Composers 28 Views Image from: khoapham.vn 29 Views − Views contain the HTML served by your application and separate your controller / application logic from your presentation logic. − Views are stored in the resources/views directory. Hello, {{ $name }} − This view is stored at resources/views/greeting.blade.php Route::get('/', function () { return view('greeting', ['name' => 'James']); }); 30 Route::get('/', function () { return view('greeting', ['name' => 'James']); }); − The first argument: the name of the view. − The second argument is an array of data that should be made available to the view. ❖ Views may also be nested within sub-directories of the resources/views directory. • For example, if your view is stored at resources/views/admin/profile.blade.php, you may reference it like so: return view('admin.profile', $data); 31 Determining If A View Exists − If you need to determine if a view exists, you may use the View facade. The exists method will return true if the view exists: use Illuminate\Support\Facades\View; if (View::exists ( 'emails.customer' )) { // } 32 Passing Data To Views − An array of data to views: return view('greetings', [ 'name' => 'Victoria', 'job'=>'Developer, 'more_data'=> $data ]); − Data should be an array with key/value pairs. • Inside your view, you can then access each value using its corresponding key, such as . • You may use the with method to add individual pieces of data to the view: return view('greeting')->with('name', 'Victoria'); 33 Sharing Data With All Views − Share a piece of data with all views that are rendered by your application. • Using the view facade's share method within a service provider's boot method. • Add them to the AppServiceProvider. namespace App\Providers; use Illuminate\Support\ServiceProvider; class AppServiceProvider extends ServiceProvider{ public function boot() { View::share('key', 'value'); } public function register() { // } } 34 Sharing Data With All Views - Example − Step 1 − Add the following line in routes/web.php Route::get('/test', function(){ return view('test'); }); Route::get('/test2', function(){ return view('test2'); }); − Step 2 − Create two view files — test.php and test2.php with the same code. These are the two files which will share data. resources/views/test.php & resources/views/test2.php 35 Sharing Data With All Views - Example − Step 3 − Change the code of boot method in the file app/Providers/AppServiceProvider.php as shown below. − (Here, we have used share method and the data that we have passed will be shared with all the views.) − app/Providers/AppServiceProvider.php namespace App\Providers; use Illuminate\Support\ServiceProvider; class AppServiceProvider extends ServiceProvider{ public function boot(){ view()->share('name', 'Nguyễn Trần Lê'); } public function register() { // } } 36 Sharing Data With All Views - Example − Step 4 − Visit the following URLs. • • 37 View Composers − Callbacks or class methods that are called when a view is rendered. − For this example: • Let's register the view composers within a service provider. • We'll use the View facade to access Illuminate\Contracts\View\Factory. • Laravel does not include a default directory for view composers. • For example, you could create an App\Http\ViewComposers directory: 38 View Composers − Create an App\Http\ViewComposers directory: namespace App\Providers; use Illuminate\Support\Facades\View; use Illuminate\Support\ServiceProvider; class ComposerServiceProvider extends ServiceProvider { public function boot() { // Using class based composers... View::composer ( 'profile', 'App\Http\ViewComposers\ProfileComposer' ); // Using Closure based composers... View::composer ( 'dashboard', function ($view) { // } ); } public function register() { // } } 39 View Composers − Now that we have registered the composer, − The ProfileComposer@compose method will be executed each time the profile view is being rendered: namespace App\Http\ViewComposers; use Illuminate\View\View; use App\Repositories\UserRepository; class ProfileComposer{ protected $users; public function __construct(UserRepository $users){ // Dependencies automatically resolved by service container... $this->users = $users; } public function compose(View $view) { $view->with('count', $this->users->count()); } } 40 Attaching A Composer To Multiple Views − Attach a view composer to multiple views: • the first argument to the composer method: View::composer( ['profile', 'dashboard'], 'App\Http\ViewComposers\MyViewComposer' ); − The * character as a wildcard, attach a composer to all views: View::composer('*', function ($view) { // }); 41 View Creators − View creators are very similar to view composers; − They are executed immediately after the view is instantiated instead of waiting until the view is about to render. View::creator('profile', 'App\Http\ViewCreators\ProfileCreator'); 42 BLADE TEMPLATES 1. Introduction 2. Template Inheritance ▪ Defining A Layout ▪ Extending A Layout 3. Displaying Data ▪ Blade & JavaScript Frameworks 4. Control Structures ▪ If Statements ▪ Loops ▪ The Loop Variable ▪ Comments ▪ PHP 5. Including Sub-Views ▪ Rendering Views For Collections 6. Stacks 43 BLADE TEMPLATES Image from: khoapham.vn 44 Giới thiệu Blade Templates − Cho phép sử dụng code PHP thuần ở trong view. − Các Blade view được compiled từ code PHP và được cache cho đến khi chúng được chỉnh sửa => không làm tăng thêm bộ nhớ. − Sử dụng đuôi .blade.php • Lưu trong resources/views. 45 Layout 2 lợi ích khi sử dụng Blade: template inheritance và sections. App Name - @yield('title') @section('sidebar') This is the master sidebar. @show @yield('content') @yield('title') is used to display the value of the title @section('sidebar') is used to define a section named sidebar @show is used to display the contents of a section @yield('content') is used to display the contents of content 46 Kế thừa một layout − Khi tạo một trang con, sử dụng Blade @extends directive để chỉ ra layout của trang con này "inherit" từ đâu. − Views kế thừa một Blade layout có thể inject nội dung vào trong sections using @section directives của layout. @extends('layouts.app') @section('title', 'Page Title') @section('sidebar') @parent This is appended to the master sidebar. @endsection @section('content') This is my body content. @endsection 47 Kế thừa một layout − Blade views có thể được trả về từ routes bằng cách sử dụng hàm global view Route::get('blade', function () { return view('child'); }); 48 @extends('app') App Name - @yield('title') @section('title', 'Page Title') @section('sidebar') @section('sidebar') @parent This is the master sidebar. @show This is appended to the master sidebar. @endsection @yield('content') @section('content') This is my body content. @endsection Route::get ( 'blade', function () { return view ( 'child' ); } ); 49 Hiển thị dữ liệu − Truyền dữ liệu vào Blade views bằng cách đặt biến trong cặp ngoặc nhọn. Route::get ( 'greeting', function () { return view ( 'welcome', [ 'name' => 'Samantha' ] ); } ); − Hiển thị nội dung của biến name variable như sau: Hello, {{ $name }} 50 Hiển thị dữ liệu nếu tồn tại − Cú pháp kiểm tra biến: {{ isset($name) ? $name : 'Default' }} − Hoặc: {{ $name or 'Default' }} 51 Hiện dữ liệu chưa Unescaped − Mặc định, cặp {{ }} được tự động gửi qua hàm htmlentities của PHP để ngăn chặn tấn công XSS. − Nếu không muốn dữ liệu bị escaped, sử dụng cú pháp: Hello, {!! $name !!}. Hello, {{ $name }}. 52 Blade & JavaScript Frameworks − Vì nhiều JavaScript frameworks cũng sử dụng cặp "ngoặc nhọn" để cho biết một biểu thức cần được hiển thị lên trình duyệt. ➢ Có thể sử dụng biểu tượng @ để nói cho Blade biết được biểu thức này cần được giữ lại. Laravel Hello, @{{ name }}. 53 The @verbatim Directive − Nếu muốn hiển thị biến JavaScript trong template ➔ Bọc chúng trong directive @verbatim Hello, {{ name }}. 54 Cấu trúc điều kiện − Cấu trúc if: • @if, @elseif, @else, và @endif. @if (count($records) === 1) I have one record! @elseif (count($records) > 1) I have multiple records! @else I don't have any records! @endif 55 Vòng lặp @for ($i = 0; $i < 10; $i++) The current value is {{ $i }} @endfor @foreach ($users as $user) This is user {{ $user->id }} @endforeach @while (true) I'm looping forever. @endwhile 56 Continue @foreach ($users as $user) @if ($user->type == 1) @continue @endif {{ $user->name }} @if ($user->number == 5) @break @endif @endforeach 57 Continue @foreach ($users as $user) @continue($user->type == 1) {{ $user->name }} @break($user->number == 5) @endforeach 58 Biến vòng lặp − Trong vòng lặp: • Một biến $loop sẽ tồn tại bên trong vòng lặp. • Cho phép truy cập một số thông tin hữu ích của vòng lặp như index của vòng lặp hiện tại, vòng lặp đầu, vòng lặp cuối @foreach ($users as $user) @if ($loop->first) This is the first iteration. @endif @if ($loop->last) This is the last iteration. @endif This is user {{ $user->id }} @endforeach 59 Biến vòng lặp − Nếu vòng lặp lồng nhau, truy cập biến $loop của vòng lặp tra qua thuộc tính parent: @foreach ($users as $user) @foreach ($user->posts as $post) @if ($loop->parent->first) This is first iteration of the parent loop. @endif @endforeach @endforeach 60 Biến $loop Thuộc tính Miêu tả $loop->index Chỉ số index hiện tại của vòng lặp (starts at 0). $loop->iteration Các vòng lặp hiện tại (starts at 1). $loop->remaining Số vòng lặp còn lại. $loop->count Tổng số vòng lặp. $loop->first Vòng lặp đầu tiên. $loop->last Vòng lặp cuối cùng. $loop->depth Độ sâu của vòng lặp hiện tại. Biến parent loop của vòng lặp trong 1 vòng lặp $loop->parent lồng. 61 Comments − Blade cho phép comment trong view. {{-- This comment will not be present in the rendered HTML --}} 62 Including Sub-Views − @include: chèn một Blade view từ một view khác. − Tất cả các biến tồn tại trong view cha đều có thể sử dụng ở view chèn thêm. @include('shared.errors') − Truyền một mảng dữ liệu bổ sung cho view @include('view.name', ['some' => 'data']) 63 Rendering Views cho Collections − Có thể kết hợp vòng lặp và view chèn thêm trong một dòng với @each directive @each('view.name', $jobs, 'job') • Tham số thứ nhất là tên của view partial để render các element trong mảng hay collection. • Tham số thứ hai là một mảng hoặc collection mà bạn muốn lặp • Tham số thứ ba là tên của biến được gán vào trong vòng lặp bên view. 64 Stacks − Để xác định thư viện JavaScript libraries cần cho view con: • Blade cho phép đẩy tên stack để cho việc render ở một vị trí nào trong view hoặc layout khác. @push('scripts') @endpush − Có thể đẩy một hoặc nhiều vào stack. @stack('scripts') 65 Mở rộng Blade − Tùy biến directives bằng phương thức directive. Khi trình viên dịch của Blade gặp directive, nó sẽ gọi tới callback được cung cấp với tham số tương ứng. namespace App\Providers; use Illuminate\Support\Facades\Blade; use Illuminate\Support\ServiceProvider; class AppServiceProvider extends ServiceProvider { public function boot() { Blade::directive('datetime', function($expression) { return "format('m/d/Y H:i'); ?>"; }); } public function register() { // } } 66 Forms & HTML − Laravel provides various in built tags to handle HTML forms easily and securely. − All the major elements of HTML are generated using Laravel. − To support this, we need to add HTML package to Laravel using composer. 67 Forms & HTML - Instalation − Begin by installing this package through Composer. Run the following from the terminal: composer require "laravelcollective/html":"^5.3.0" − Next, add your new provider to the providers array of config/app.php: 'providers' => [ // ... Collective\Html\HtmlServiceProvider::class, // ... ], − Finally, add two class aliases to the aliases array of config/app.php: 'aliases' => [ // ... 'Form' => Collective\Html\FormFacade::class, 'Html' => Collective\Html\HtmlFacade::class, // ... ], 68 Forms & HTML - Opening A Form {!! Form::open(['url' => 'foo/bar']) !!} // {!! Form::close() !!} − By default, a POST method will be assumed; however, you are free to specify another method: echo Form::open(['url' => 'foo/bar', 'method' => 'put']) − Note: Since HTML forms only support POST and GET, PUT and DELETE methods will be spoofed by automatically adding a _method hidden field to your form. 69 Forms & HTML - Opening A Form − You may also open forms that point to named routes or controller actions: echo Form::open(['route' => 'route.name']) echo Form::open(['action' => 'Controller@method']) − You may pass in route parameters as well: echo Form::open(['route' => ['route.name', $user->id]]) echo Form::open(['action' => ['Controller@method', $user->id]]) − If your form is going to accept file uploads, add a files option to your array: echo Form::open(['url' => 'foo/bar', 'files' => true]) 70 Forms & HTML - Label − Generating A Label Element echo Form::label('email', 'E-Mail Address'); − Specifying Extra HTML Attributes echo Form::label('email', 'E-Mail Address', ['class' => 'awesome']); − Note: After creating a label, any form element you create with a name matching the label name will automatically receive an ID matching the label name as well. 71 Forms & HTML - Text Input − Generating A Text Input echo Form::text('username'); − Specifying A Default Value echo Form::text('email', 'example@gmail.com'); − Note: The hidden and textarea methods have the same signature as the text method. 72 Forms & HTML - Password Input − Generating A Password Input echo Form::password('password', ['class' => 'awesome']); − Generating Other Inputs echo Form::email($name, $value = null, $attributes = []); echo Form::file($name, $attributes = []); 73 Forms & HTML - Checkbox Or Radio Input − Generating A Checkbox Or Radio Input echo Form::checkbox('name', 'value'); echo Form::radio('name', 'value'); − Generating A Checkbox Or Radio Input That Is Checked echo Form::checkbox('name', 'value', true); echo Form::radio('name', 'value', true); 74 Forms & HTML – Number, Date, File − Generating A Number Input echo Form::number('name', 'value'); − Generating A Date Input echo Form::date('name', \Carbon\Carbon::now()); − Generating A File Input echo Form::file('image'); 75 Forms & HTML – Drop-Down Lists − Generating A Number Input echo Form::number('name', 'value'); − Generating A Drop-Down List echo Form::select('size', ['L' => 'Large', 'S' => 'Small']); − Generating A Drop-Down List With Selected Default echo Form::select('size', ['L' => 'Large', 'S' => 'Small'], 'S'); − Generating a Drop-Down List With an Empty Placeholder • This will create an element with no value as the very first option of your drop- down. echo Form::select('size', ['L' => 'Large', 'S' => 'Small'], null, ['placeholder' => 'Pick a size...']); 76 Forms & HTML – Drop-Down Lists − Generating a List With Multiple Selectable Options echo Form::select('size', ['L' => 'Large', 'S' => 'Small'], null, ['multiple' => true]); − Generating A Grouped List echo Form::select('animal',[ 'Cats' => ['leopard' => 'Leopard'], 'Dogs' => ['spaniel' => 'Spaniel'], ]); − Generating A Drop-Down List With A Range echo Form::selectRange('number', 10, 20); − Generating A List With Month Names − echo Form::selectMonth('month'); 77 Forms & HTML – Buttons − Generating A Submit Button echo Form::submit('Click Me!'); − Note: Need to create a button element? Try the button method. It has the same signature as submit. 78 Forms & HTML – Generating URLs − Generate a HTML link to the given URL. echo link_to('foo/bar', $title = null, $attributes = [], $secure = null); − Generate a HTML link to the given asset. echo link_to_asset('foo/bar.zip', $title = null, $attributes = [], $secure = null); − Generate a HTML link to the given named route. echo link_to_route('route.name', $title = null, $parameters = [], $attributes = []); − Generate a HTML link to the given controller action. echo link_to_action('HomeController@getIndex', $title = null, $parameters = [], $attributes = []); 79 Forms & HTML – Example − resources/views/form.php <?php echo Form::open(array('url' => 'foo/bar')); echo Form::text('username','nguyentranle') . ''; echo Form::text('email', 'nguyentranle@gmail.com') . ''; echo Form::password('password') . ''; echo Form::checkbox('name', 'value') . 'Checkbox'; echo Form::radio('name', 'value') . 'Radio button'; echo Form::file('image') . ''; echo Form::select('size', array('L' => 'Large', 'S' => 'Small')) . ''; echo Form::submit('Click Me!'); echo Form::close(); ?> 80 Forms & HTML – Example − Routes/web.php Route::get('/form',function(){ return view('form'); }); − Test: 81
File đính kèm:
bai_giang_phat_trien_phan_mem_nguon_mo_bai_7_route_views_bla.pdf